Excel VBA-strengesammenligning
For at sammenligne to strenge i VBA har vi en indbygget funktion, dvs. “ StrComp ”. Dette kan vi læse som " String Comparison ", denne funktion er kun tilgængelig med VBA og er ikke tilgængelig som en regnearkfunktion. Den sammenligner to strenge og returnerer resultaterne som "Nul (0)", hvis begge strengene matcher, og hvis begge leverede strenge ikke stemmer overens, får vi "En (1)" som resultat.
I VBA eller excel står vi over for masser af forskellige scenarier. Et sådant scenario er "sammenligning af to strengværdier." I et almindeligt regneark kan vi gøre disse flere måder, men i VBA, hvordan gør du dette?

Nedenfor er syntaksen for “StrComp” -funktionen.

For det første er to argumenter ganske enkle,
- for streng 1 skal vi levere, hvad den første værdi vi sammenligner, og
- for streng 2 skal vi levere den anden værdi, vi sammenligner.
- (Sammenlign) dette er det valgfri argument for StrComp-funktionen. Dette er nyttigt, når vi vil sammenligne store og små bogstaver. For eksempel er "Excel" i dette argument ikke lig med "EXCEL", fordi begge disse ord er store og små bogstaver.
Vi kan levere tre værdier her.
- Nul (0) for " Binær sammenligning ", dvs. "Excel", er ikke lig med "EXCEL." Til sammenligning af store og små bogstaver kan vi levere 0.
- Én (1) for " Tekstsammenligning ", dvs. "Excel", er lig med "EXCEL." Dette er en ikke-sagsfølsom sammenligning.
- To (2) dette kun til databasesammenligning.
Resultaterne af "StrComp" -funktionen er ikke standard SAND eller FALSK, men varierer. Nedenfor er de forskellige resultater af “StrComp” -funktionen.
- Vi får “0” som resultat, hvis de medfølgende strenge matcher.
- Vi får “1”, hvis de medfølgende strenge ikke stemmer overens, og i tilfælde af numerisk matching får vi 1, hvis streng 1 er større end streng 2.
- Vi får “-1”, hvis streng 1 nummer er mindre end streng 2 nummer.
Hvordan udføres strengesammenligning i VBA?
Eksempel nr. 1
Vi matcher " Bangalore " mod strengen " BANGALORE ."
Først skal du erklære to VBA-variabler som strengen til at gemme to strengværdier.
Kode:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String End Sub

For disse to variabler skal du gemme to strengværdier.
Kode:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 Som String Value1 = "Bangalore" Value2 = "BANGALORE" End Sub
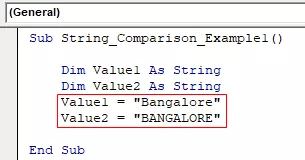
Erklær endnu en variabel for at gemme resultatet af “ StrComp ” -funktionen.
Kode:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String End Sub

Åbn “StrComp” -funktionen for denne variabel.
Kode:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp (End Sub
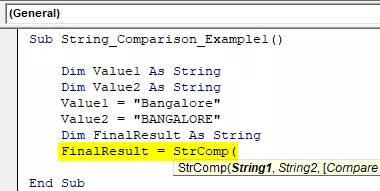
For “String1” & “String2” har vi allerede tildelt værdier gennem variabler, så indtast henholdsvis variabelnavne.
Kode:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp (Value1, Value2, End Sub
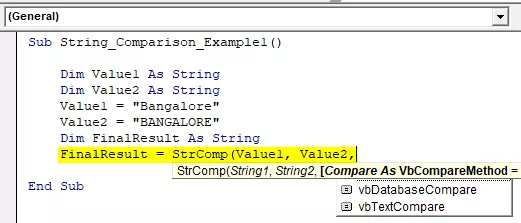
Den sidste del af funktionen er "Sammenlign" for dette valg "vbTextCompare."
Kode:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp (Value1, Value2, vbTextCompare) End Sub

Vis nu variablen "Endeligt resultat" i meddelelsesfeltet i VBA.
Kode:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 Som String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp (Value1, Value2, vbTextCompare) MsgBox FinalResult End Sub Sub

Ok, lad os køre koden og se resultatet.
Produktion:

Da begge strengene "Bangalore" og "BANGALORE" er de samme, fik vi resultatet som 0, dvs. matching. Begge værdier er store og små bogstaver, da vi har leveret argumentet som “vbTextCompare”, det har ignoreret store og små bogstaver og matchede kun værdier, så begge værdier er de samme, og resultatet er 0, dvs. SAND.
Kode:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 Som String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp (Value1, Value2, vbTextCompare) MsgBox FinalResult End Sub Sub

Eksempel 2
For the same code, we will change the compare method from “vbTextCompare” to “vbBinaryCompare.”
Code:
Sub String_Comparison_Example2() Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Now run the code and see the result.
Output:

Even though both the strings are the same, we got the result as 1, i.e., Not Matching because we have applied the compare method as “vbBinaryCompare,” which compares two values as case sensitive.
Example #3
Now we will see how to compare numerical values. For the same code, we will assign different values.
Code:
Sub String_Comparison_Example3() Dim Value1 As String Dim Value2 As String Value1 = 500 Value2 = 500 Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Both the values are 500, and we will get 0 as a result because both the values are matched.
Output:
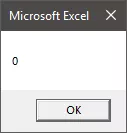
Now I will change the Value1 number from 500 to 100.
Code:
Sub String_Comparison_Example3() Dim Value1 As String Dim Value2 As String Value1 = 1000 Value2 = 500 Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Run the code and see the result.
Output:
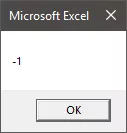
We know Value1 & Value2 aren’t the same, but the result is -1 instead of 1 because for numerical comparison when the String 1 value is greater than String 2, we will get this -1.
Code:
Sub String_Comparison_Example3() Dim Value1 As String Dim Value2 As String Value1 = 1000 Value2 = 500 Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Now I will reverse the values.
Code:
Sub String_Comparison_Example3() Dim Value1 As String Dim Value2 As String Value1 = 500 Value2 = 1000 Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Run the code and see the result.
Output:

This is not special. If not match, we will get 1 only.
Things to Remember here
- (Compare) argument of “StrComp” is optional, but in case of case sensitive match, we can utilize this, and the option is “vbBinaryCompare.”
- The result of numerical values is slightly different in case String 1 is greater than string 2, and the result will be -1.
- Results are 0 if matched and 1 if not matched.
Recommended Articles
Dette har været en guide til VBA-strengesammenligning. Her diskuterer vi, hvordan man sammenligner to strengværdier ved hjælp af StrComp-funktionen i excel VBA sammen med eksempler og downloader en excel-skabelon. Du kan også se på andre artikler relateret til Excel VBA -
- Vejledning til VBA-strengfunktioner
- VBA Split streng i Array
- VBA SubString-metoder
- VBA-tekst